Introduction
Playwright is a powerful automation framework developed by Microsoft that enables end-to-end testing for web applications. It supports multiple browsers, including Chromium, Firefox, and WebKit, and works seamlessly across different operating systems. Playwright is widely used for automated UI testing, web scraping, and performance monitoring.
Why Choose Playwright?
- Cross-browser Support – Test applications in multiple browsers without modifying scripts.
- Fast Execution – Uses a single API for automation, ensuring quicker test execution.
- Headless Mode – Run tests without opening a browser UI, improving speed.
- Auto-wait Mechanism – Reduces flaky tests by waiting for elements before interacting with them.
- Built-in Report Generation – Generate test reports for better analysis and debugging.
- Multi-language Support – Works with JavaScript, TypeScript, Python, Java, and C#.
Setting Up Playwright
1. Installing Playwright
Playwright can be installed using npm, yarn, or pip, depending on your preferred language.
For JavaScript/TypeScript:
npm init playwright@latest
For Python:
pip install playwright playwright install
2. Launching a Browser
A simple script to launch a browser using Playwright:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://example.com');
await browser.close();
})();
Writing Your First Test
Playwright supports modern testing frameworks like Jest and Playwright Test. Here’s an example test:
const { test, expect } = require('@playwright/test');
test('Check page title', async ({ page }) => {
await page.goto('https://example.com');
await expect(page).toHaveTitle('Example Domain');
});
Advanced Playwright Features
1. Handling Authentication
Playwright can automate login processes and persist authentication state:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
await page.goto('https://example.com/login');
await page.fill('#username', 'testuser');
await page.fill('#password', 'password');
await page.click('button[type=submit]');
await context.storageState({ path: 'auth.json' });
await browser.close();
})();
2. Parallel Test Execution
Playwright allows running tests in parallel for faster execution:
npx playwright test --shard=3/3
3. Capturing Screenshots and Videos
Playwright can take screenshots and record videos for debugging:
await page.screenshot({ path: 'screenshot.png' });
await page.video().saveAs('video.mp4');
Debugging and Reporting
- Debug Mode:
npx playwright test --debug
- Tracing Execution:
npx playwright test --trace on
- Generating Reports:
npx playwright show-report
Conclusion
Playwright is a robust and reliable automation tool that simplifies web testing and automation. Its extensive features, ease of use, and cross-browser compatibility make it an excellent choice for developers and testers. By leveraging Playwright, teams can enhance their testing capabilities, improve efficiency, and ensure high-quality web applications.
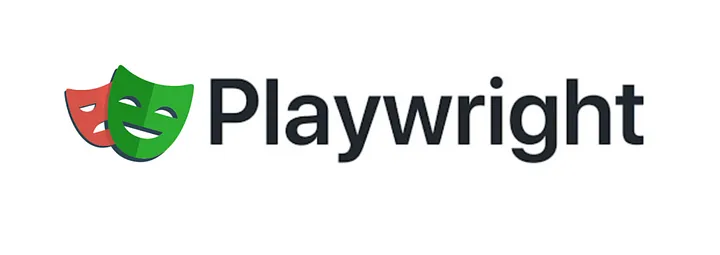