Learn how to use Playwright and Cucumber to automate browser testing
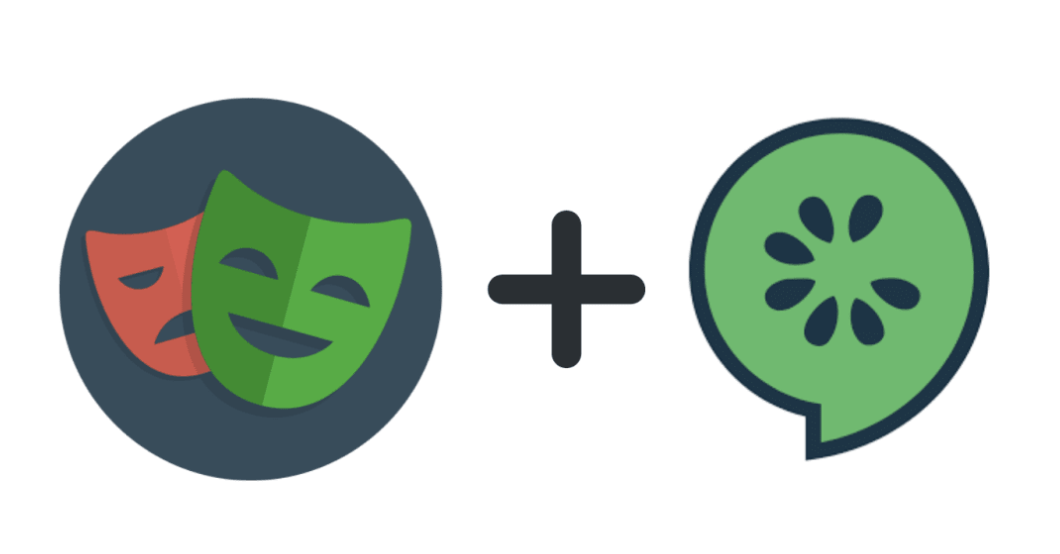
Learn how to use Playwright and Cucumber to automate browser testing
Overview
What is Playwright?
Playwright is a powerful open-source library for automating web browsers. It provides a high-level API to control browsers such as Chrome, Firefox, and WebKit, allowing you to automate tasks like navigating, clicking buttons, filling forms, and capturing screenshots.
Unlike other browser automation frameworks, Playwright supports multiple browser engines, allowing you to write cross-browser tests. This means you can write your automated test scripts once and run them across different browsers to ensure your application functions correctly in all popular browsers.
Key Features
Playwright offers several key features that make it a preferred choice for browser automation:
1. Cross-Browser Compatibility: Playwright supports the Chrome, Firefox, and WebKit browser engines. This allows you to write tests that can be executed on multiple browsers, ensuring consistent behavior across different platforms.
2. Multi-Page and Multi-Context Support: With Playwright, you can handle multiple browser pages and contexts within a single test script. This feature enables you to handle scenarios such as concurrent user sessions, pop-up windows, or multiple tabs.
3. Web and Mobile Browser Automation: Playwright allows you to automate both web and mobile browsers, making it suitable for testing web applications that are responsive or have dedicated mobile views.
4. Powerful API: Playwright provides a high-level API with a wide range of methods and selectors, allowing you to interact with web elements easily. It also supports network interception and modification, making it convenient for testing scenarios involving network requests.
5. Snapshot Testing: Playwright has built-in support for taking screenshots and videos of browser interactions, making it easy to visualize and debug test failures.
Installing Playwright
To get started with Playwright, you’ll need to install it on your system. Playwright supports Node.js, Python, and .NET, so you can choose the language that suits your needs.
Here is a brief guide to installing Playwright in Node.js:
- Open your terminal or command prompt.
- Run the following command to install Playwright:
npm install playwright
. - Once the installation is complete, you can start using Playwright in your Node.js projects.
Writing Your First Playwright Test
Now that you have Playwright installed, let’s write a simple test to get familiar with the framework.
-
Create a new file called
example.test.js
in your project directory. -
Import Playwright at the beginning of the file using the following code:
const { chromium } = require('playwright');
-
Define an async function to run your test:
(async () => { // Your test code goes here })();
-
Instantiate a new browser context and page:
const browser = await chromium.launch(); const context = await browser.newContext(); const page = await context.newPage();
-
Navigate to a webpage:
await page.goto('https://www.example.com');
-
Perform actions on the web page:
await page.click('button'); await page.fill('input[name="username"]', 'testuser');
-
Capture a screenshot:
await page.screenshot({ path: 'example.png' });
-
Close the browser:
await browser.close();
-
Run the test script using Node.js:
node example.test.js
Congratulations! You’ve written and executed your first Playwright test. This simple example demonstrates the basic capabilities of Playwright and how you can automate browser interactions using its powerful API.
Cucumber is a widely used testing tool that allows for behavior-driven development (BDD) in software development projects. It provides a simple and easily readable way to define and execute test scenarios using the Gherkin language.
What is Cucumber?
Cucumber is an open-source tool that facilitates collaboration between developers, testers, and stakeholders by enabling them to express the desired behavior of an application using plain English. It allows non-technical individuals to write test scenarios and helps bridge the communication gap between technical and non-technical team members.
Benefits of Cucumber
Using Cucumber in your development process offers a range of benefits:
1. Collaboration: Cucumber promotes collaboration between team members with different skill sets. It allows individuals to define and understand the behavior of the software using a common language.
2. Readability: By using the Gherkin language, Cucumber makes tests readable to both technical and non-technical team members. This improves the overall understanding of the system’s behavior.
3. Reusability: Cucumber promotes the reuse of step definitions across different test scenarios. This allows teams to save time and effort by avoiding duplication of code.
4. Test Documentation: Cucumber test scenarios serve as live documentation for the system’s behavior. They provide clear, concise examples that can be understood by both technical and non-technical team members.
5. Test Driven Development (TDD): Cucumber supports TDD by allowing teams to write tests before implementing the code. This approach helps in better understanding the requirements and ensures that the code meets those requirements.
Gherkin Language
Cucumber uses the Gherkin language to describe test scenarios in a structured and human-readable format. Gherkin consists of a set of keywords that define the steps involved in a scenario. These keywords include Given, When, Then, And, and But.
The Gherkin syntax allows you to define preconditions (Given), actions (When), expected outcomes (Then), and additional steps (And/But) for a given scenario. This structured approach ensures clarity and maintainability of the test scenarios.
Cucumber Features and Scenarios
Cucumber organizes test scenarios into features and scenarios based on the functionality and behavior of the application.
A feature represents a specific aspect of the application and contains one or more scenarios. It provides a high-level description of the behavior being tested.
A scenario represents a specific test case within a feature and consists of a sequence of steps defined using Gherkin keywords. Each scenario focuses on a particular behavior or functionality and provides clear steps to reproduce and verify it.
Cucumber Execution
Cucumber uses a test runner to execute the defined test scenarios. The test runner reads the feature files and executes the corresponding step definitions based on the defined keywords. Step definitions are written in a programming language such as JavaScript, Java, or Ruby, depending on the project’s requirements.
During execution, Cucumber matches each step in a scenario with its corresponding step definition. If a matching step definition is found, the associated code is executed. Cucumber provides hooks that allow developers to perform additional setup and teardown actions before and after scenarios or steps.
Integration of Playwright and Cucumber
Playwright is an open-source automation tool that allows developers and testers to write browser tests using JavaScript or TypeScript. On the other hand, Cucumber is a tool that enables the execution of behavior-driven development (BDD) tests in a readable and understandable format. In this topic, we will explore how to integrate Playwright and Cucumber to create efficient and maintainable browser automation tests.
Why integrate Playwright and Cucumber?
By combining Playwright and Cucumber, we can leverage the strengths of both tools to create more powerful and expressive tests. Playwright provides a robust framework for automating browser actions, while Cucumber offers a natural language syntax for describing test scenarios. The integration of these two tools allows for enhanced test readability, reusability, and collaboration among team members.
Setting up Playwright and Cucumber integration
To start using Playwright and Cucumber together, you need to install both tools and configure your project accordingly. Begin by installing Node.js, which is required by both Playwright and Cucumber. Once Node.js is installed, you can proceed with installing Playwright and Cucumber as dependencies using the Node Package Manager (npm).
Next, you will need to create a Cucumber configuration file that specifies the desired Playwright browser context and other relevant settings. This configuration file, commonly named cucumber.ts
or cucumber.js
, allows you to define global hooks, generate reports, and set up the environment for your tests.
Writing feature files with Playwright and Cucumber
With Playwright and Cucumber integrated, you can start writing feature files that describe the desired behavior of your software. These feature files follow the Gherkin syntax, which provides a structured format for specifying test scenarios using human-readable language.
Within a feature file, you define scenarios using the Given
, When
, and Then
keywords. Each step in these scenarios corresponds to a specific action or assertion to be performed in the browser. Playwright’s comprehensive API can be used within Cucumber step definitions to interact with the browser, such as navigating to URLs, interacting with forms, or asserting element properties.
By utilizing Cucumber’s data-driven capabilities, you can further enhance the flexibility of your tests. Cucumber allows you to use tables or regular expressions within your feature files to parameterize your steps and run them with different inputs or datasets.
Executing Playwright and Cucumber tests
To run tests written with the Playwright and Cucumber integration, simply execute the Cucumber command with the appropriate arguments. This command triggers the execution of the feature files, which in turn invokes the underlying step definitions and actions specified using Playwright.
During the test execution, you will see a detailed output that indicates the progress and status of each step. Playwright and Cucumber provide comprehensive reporting options, allowing you to generate useful test reports in various formats, including HTML, JSON, or plain text.
Benefits of the Playwright and Cucumber integration
Integrating Playwright and Cucumber brings numerous benefits to the testing process. Firstly, the natural language syntax of Cucumber makes test scenarios more readable and accessible to non-technical team members. This improves collaboration and enables stakeholders to understand the testing process easily.
Secondly, Playwright’s powerful browser automation capabilities enable smooth and reliable interaction with web applications. The integration with Cucumber ensures maintainable and easy-to-understand tests that can be executed repeatedly, promoting robustness and more efficient use of testing resources.
Finally, the integration of Playwright and Cucumber facilitates the adoption of behavior-driven development practices, enabling teams to incorporate testing early in the software development lifecycle. By aligning test scenarios with user behaviors, it becomes easier to verify that the software meets the expected requirements.